App Development Philadelphia
App Developers Philadelphia, PA
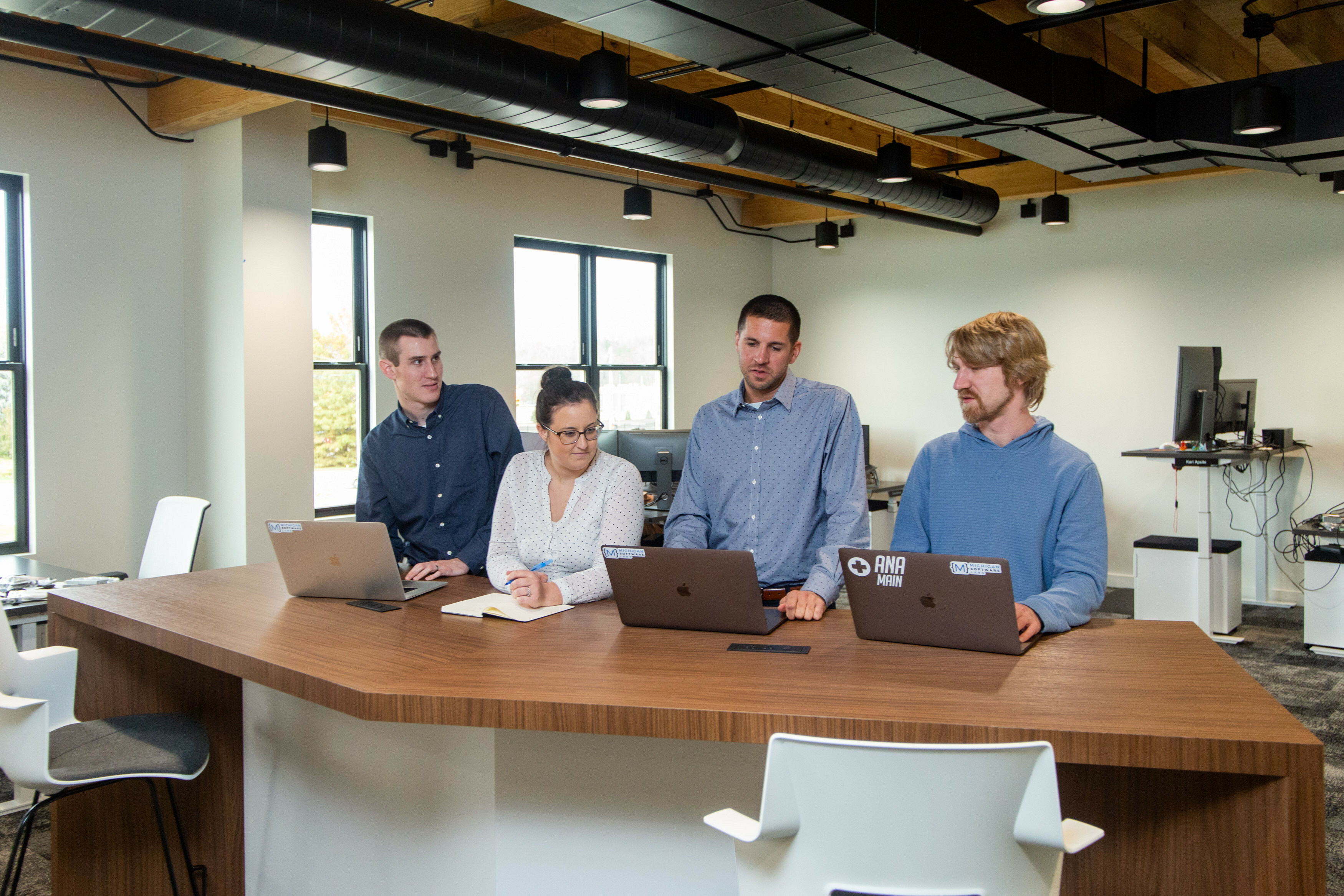
Does your company need to develop an app? Let’s start with the basics of app development.
- Ideation
- Design
- Execution
Michigan Software Labs has app developers providing a complete turnkey solution to those looking to turn their mobile app dreams into reality. Our team of developers and designers can guide you through the twists and turns of app development, simplifying an often convoluted and complex process.
We can develop a customized iPhone or Android app solution to meet your specifications and scale accordingly to satisfy user demands.
Philadelphia iPhone and Android app developers. We work with many different types of companies and can assist you in every phase of the mobile app development process. From fleshing out an idea to submitting a finalized app into the App Store or Play Store, we have the experience to make your digital product strategy become a reality.
How we work
App Idea to Rapid Prototyping
User Experience (UX) and User Interface (UI) Design
The Programming Process
Support after Go-live
Every app begins with an idea. We’ll help you find the answers to three fundamental questions you need to consider before moving forward with your idea:
Who are the users of your app?
What will your app do?
What value will this app create?
Why will someone use this app?
Answering these questions will bring your idea into focus, allowing you to move forward with confidence. At Michigan Software Labs, we use storyboards and wireframes to help clients conceive and refine their ideas, moving them into the realm of reality.
"We Love Working With Our Clients"
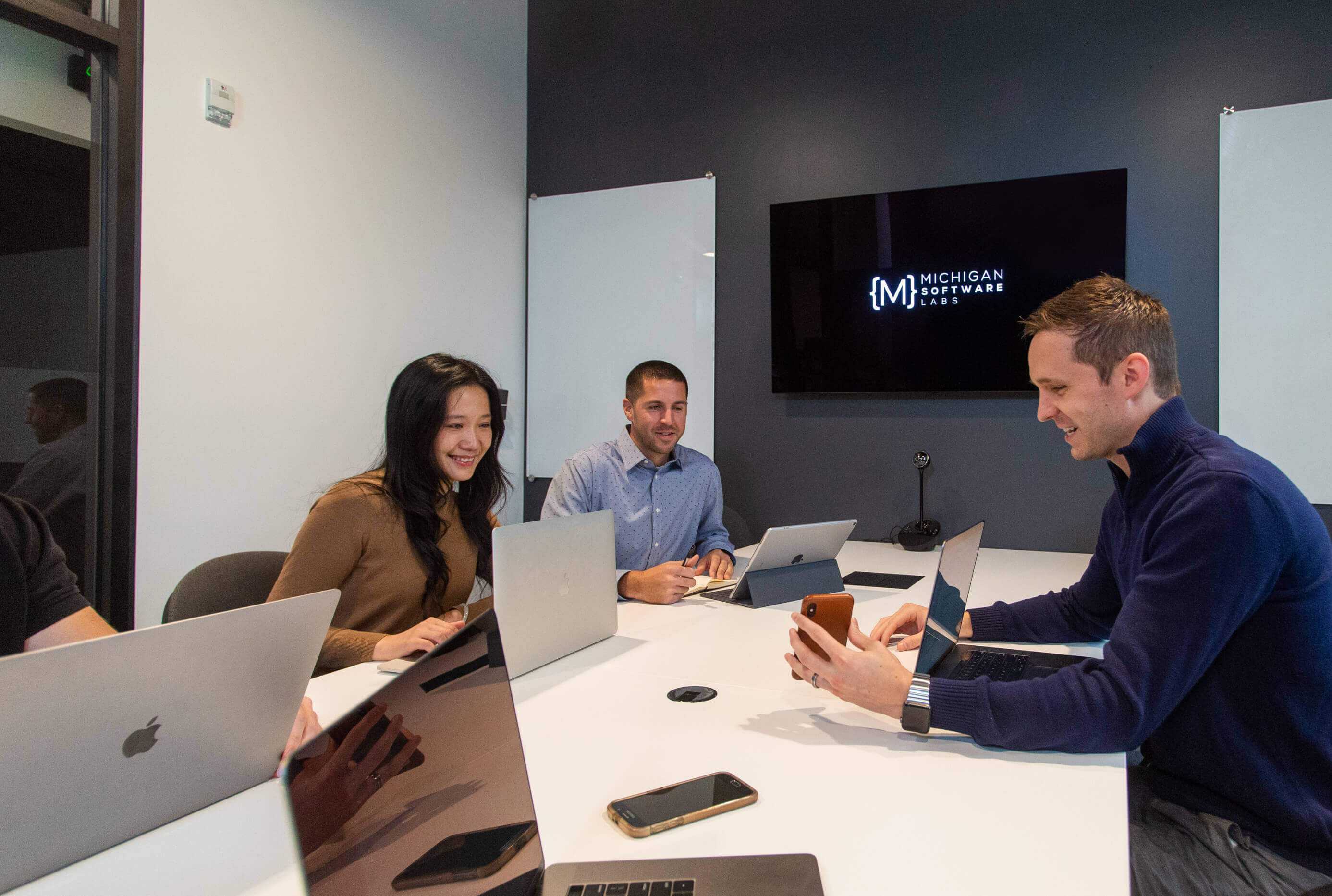
Who we are
Michigan Software Labs is a mobile app development company which creates apps to solve large problems for large companies. The team develops custom mobile, web, and Internet of Things (IoT) software for clients ranging from locally owned businesses to Fortune 500 companies. Recognized as a leader in UX/UI design, with millions of end users to date, Michigan Software Labs was named one of the Top UX Design Companies in the U.S. by Clutch in 2018 and the Inc. Best Places to Work Award in 2019.
Our Process
1. DISCOVER AND ARCHITECT
Aligning business, technology, and customer needs.
Business discovery
User research
Cloud architecture
Information Architecture
Innovation workshops
Business and Insights
Data opportunity strategy
Feature roadmap prioritization
DISCOVERY AND ARCHITECTURE AT MICHIGAN SOFTWARE LABS IS ALL ABOUT SERVING OUR CLIENTS
Innovation doesn’t happen around a boardroom table. That’s why we employ proven methods that put us in the shoes of your users. At Michigan Software Labs, human-centered research always drives design and development.
2. DESIGN & PROTOTYPE
Human-centered product design & prototyping
User experience
Visual design
Customer journey mapping
Interaction design
Wireframes
Interactive prototypes
Content design
Design systems
Voice UI & chatbot design
Conceptual design
User testing
DESIGN & PROTOTYPING AT MICHIGAN SOFTWARE LABS
We take pride in our craft. Drawing on our deep expertise in design, our Product Design team cares for your user’s experience over the entire customer journey, at every touchpoint with your company.
3. BUILD & DEPLOY
Carefully crafted stack & continuously released code
Front-end development
Back-end development
Web service development
DevOps
Automated testing
Systems architecture
Agile/SCRUM
API development
Front-end component libraries
MVPs & prototypes
Release management
Continuous integration
Security & compliance
DEVELOPMENT AT MICHIGAN SOFTWARE LABS
We hire the best custom app development teams (choosing from more than 100 applicants for every software development position).
Working together in co-located teams, we constantly learn from and challenge each other. Our teams stay lean and rapidly deploy customized solutions for each client.
4. SCALE & MEASURE
Analytics on business success metrics & customer nurturing
Usability Testing
Integrated analytics
KPI measurement strategy
Feature roadmapping
Ongoing maintenance
Cloud optimization
Release planning
Continuous integration
A/B Testing
SCALING WITH MICHIGAN SOFTWARE LABS
We help to get your product to market. Whether that is on Apple’s App Store®, Google’s PlayStore™, or somewhere else. The objective is to make sure your app is available for your users. As your product grows and evolves, we are here to help support you. Maintaining a product may involve keeping it up-to-date with the latest operating system changes or ensuring it works on new devices.
Serving You
App Development Services, iPhone App Development, Android App Development,Healthcare App Development,Financial App Development,Machine Learning App Development, AI App Development, Internet of Things App Development
List of Geographic Areas We Hear from Most Often
app development austin |
app development arlington |
app development baltimore |
app development charlotte |
app development colorado springs |
app development columbus |
app development detroit |
app development el paso |
app development fort worth |
app development fresno |
app development jacksonville |
app development las vegas |
app development long beach |
app development louisville |
app development memphis |
app development mesa |
app development new orleans |
app development oakland |
app development oklahoma city |
app development omaha |
app development raleigh |
app development san jose |
app development tucson |
app development tulsa |
app development virginia beach |
app development washington |
app development wichita |
app development indianapolis |
app development philadelphia |
app development nashville |
app development atlanta |
app development milwaukee |
app development austin |
app development portland |
app development miami |
app development houston |
app development sacramento |
app development dallas |
app development phoenix |
app development minneapolis |
app development san diego |
app development boston |
app development san antonio |
app development los angeles |
app development chicago |
app development denver |
app development new york |
app development kansas city |
app development san francisco |
app development seattle |